main.py
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
from kivy.lang import Builder
from kivymd.app import MDApp
class MainApp(MDApp):
def build(self):
self.theme_cls.theme_style = "Dark"
# self.theme_cls.theme_style = "Light"
self.theme_cls.primary_palette = "BlueGray"
return Builder.load_file('abc.kv')
def logger(self):
self.root.ids.welcome_label.text= f'Sup {self.root.ids.user.text}!'
def clear(self):
self.root.ids.welcome_label.text ='welcome'
self.root.ids.user.text =''
self.root.ids.password.text =''
MainApp().run()
|
cs |
abc.kv
가운데 정렬 : pos_hint: {"center_x": 0.5, "center_y": 0.5} # 1 == 100%
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
|
Screen:
MDCard:
size_hint: None, None
size: 300, 400
pos_hint: {"center_x": 0.5, "center_y": 0.5}
elevation: 10
padding: 25
spacing: 25
orientation: 'vertical'
MDLabel:
id: welcome_label
text: "Welcome"
font_size: 40
halign: 'center'
size_hint_y: None
height: self.texture_size[1]
padding_y: 15
MDTextFieldRound:
id: user
hint_text: "username"
icon_right: "account"
size_hint_x: None
width: 200
font_size: 18
pos_hint: {"center_x": 0.5}
MDTextFieldRound:
id: password
hint_text: "password"
icon_right: "eye-off"
size_hint_x: None
width: 200
font_size: 18
pos_hint: {"center_x": 0.5}
password: True
MDRoundFlatButton:
text: "LOGIN"
font_size: 12
pos_hint: {"center_x": 0.5}
on_press: app.logger()
MDRoundFlatButton:
text: "clear"
font_size: 12
pos_hint: {"center_x": 0.5}
on_press: app.clear()
Widget:
size_hint_y: None
height: 10
|
cs |
main.py
1
2
3
4
5
6
7
8
9
10
11
12
13
|
from kivy.lang import Builder
from kivymd.app import MDApp
class MainApp(MDApp):
def build(self):
self.theme_cls.theme_style = "Dark"
# self.theme_cls.theme_style = "Light"
self.theme_cls.primary_palette = "BlueGray"
return Builder.load_file('abc.kv')
def presser(self):
self.root.ids.my_label.text = "hi my name is 2toy"
MainApp().run()
|
cs |
abc.kv
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
MDBoxLayout:
orientation: 'vertical'
MDToolbar:
title: "OUr Top Toolbar"
left_action_items: [["menu"]]
right_action_items: [["dots-vertical"]]
MDLabel:
id: my_label
text: "some Stuff"
halign: "center"
MDBottomAppBar:
MDToolbar:
icon: 'git'
title: "bottom Menu"
left_action_items: [["menu"]]
type: 'bottom'
#mode: "free-end"
#mode: "free-center"
mode: "end"
#mode: "center"
on_action_button: app.presser()
|
cs |
main.py
1
2
3
4
5
6
7
8
9
10
11
12
|
from kivy.lang import Builder
from kivymd.app import MDApp
class MainApp(MDApp):
def build(self):
self.theme_cls.theme_style = "Dark"
# self.theme_cls.theme_style = "Light"
self.theme_cls.primary_palette = "BlueGray"
return Builder.load_file('abc.kv')
MainApp().run()
|
cs |
abc.kv
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
|
BoxLayout:
orientation: 'vertical'
MDToolbar:
title: "Bottom Navbar"
md_bg_color: .2,.2,.2,.2
specific_text_color: 1,1,1,1
MDBottomNavigation:
#color stuff
#panel_color: 0,0,1,1
MDBottomNavigationItem:
name: 'screen 1'
text: "python"
icon: 'language-python'
MDLabel:
text: "Python"
halign: 'center'
MDBottomNavigationItem:
name: 'screen 2'
text: "Youtube"
icon: 'youtube'
MDLabel:
text: "hello youtube"
halign: 'center'
MDBottomNavigationItem:
name: 'screen 3'
text: "Instagram"
icon: 'instagram'
MDLabel:
text: "My Instagram:\nreal"
halign: 'center'
|
cs |
아이콘 정보 체크
http://zavoloklom.github.io/material-design-iconic-font/icons.html
Material Design Iconic Font - Icons List
Material Design Iconic Font is a full suite of official material design icons and community icons for easy scalable vector graphics on websites or desktop.
zavoloklom.github.io
main.py
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
|
from kivy.lang import Builder
from kivymd.app import MDApp
class MainApp(MDApp):
data = {
'python': "language-python",
'ruby': "language-ruby",
'C': "language-c"
}
def callback(self, instance):
if instance.icon == 'language-python':
lang = "Python"
elif instance.icon == 'language-ruby':
lang = "Ruby"
elif instance.icon == 'language-c':
lang = "C"
self.root.ids.my_label.text = f'you pressed {lang}'
# self.root.ids.my_label.text = f'you pressed {instance.icon}'
#Open
def open(self):
self.root.ids.my_label.text = f'open'
#Close
def close(self):
self.root.ids.my_label.text = f'close'
def build(self):
self.theme_cls.theme_style = "Dark"
# self.theme_cls.theme_style = "Light"
self.theme_cls.primary_palette = "BlueGray"
return Builder.load_file('abc.kv')
MainApp().run()
|
cs |
abc.kv
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
MDBoxLayout:
orientation: 'vertical'
MDScreen:
MDLabel:
id: my_label
text: "Some Stuff"
halign: "center"
MDFloatingActionButtonSpeedDial:
data: app.data
root_button_anim: True
#root_button_anim: False
######Color stuff#####
#label_text_color: 1,0,0,1 # Text color
#bg_color_stack_button: 1,0,0,1 # icon bg color
#bg_color_root_button: 1,0,0,1 # button bg color
#color_icon_root_button: 1,1,0,1 # speed dial text color
#color_icon_stack_button: 1,0,1,1 # icond text color
#bg_hint_color: 1,1,1,1 #icon bgcolor
callback: app.callback
#open or Close
on_open: app.open()
on_close: app.close()
|
cs |
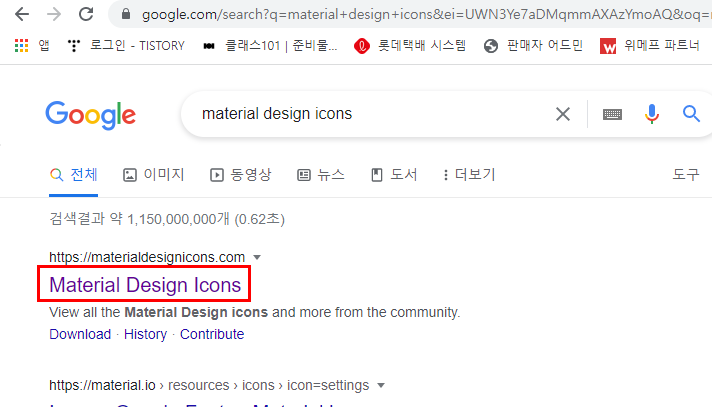
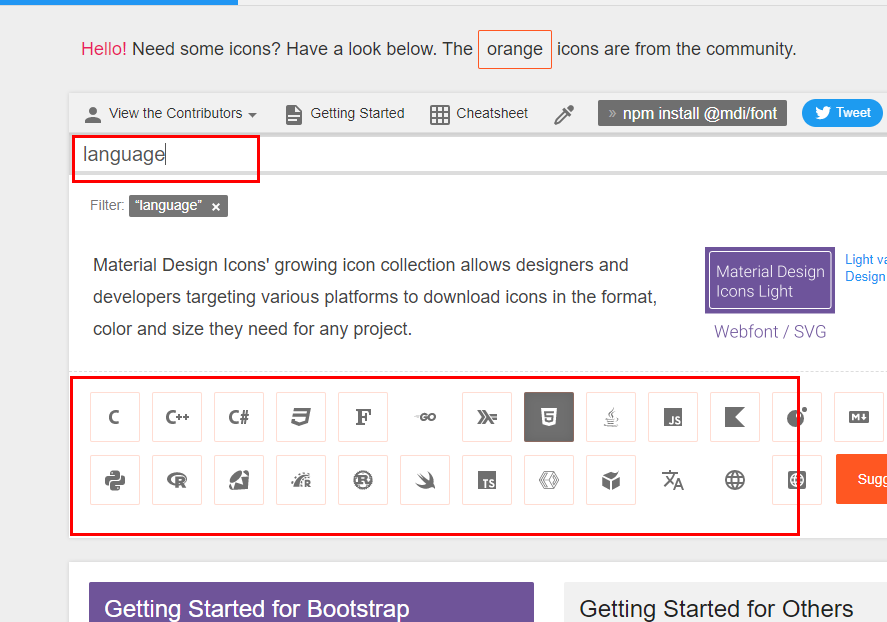
https://materialdesignicons.com/
Material Design Icons
Material Design Icons' growing icon collection allows designers and developers targeting various platforms to download icons in the format, color and size they need for any project. Loading... Sponsored by Icons8 Material Icon Sets
materialdesignicons.com
main.py
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
|
from kivy.lang import Builder
from kivymd.app import MDApp
from kivymd.uix.button import MDFlatButton, MDRectangleFlatButton
from kivymd.uix.dialog import MDDialog
class MainApp(MDApp):
dialog = None
def build(self):
self.theme_cls.theme_style = "Dark"
# self.theme_cls.theme_style = "Light"
self.theme_cls.primary_palette = "BlueGray"
return Builder.load_file('abc.kv')
def show_alert_dialog(self):
if not self.dialog:
self.dialog = MDDialog(
title = "hello !", text = "hi 2toy world",
buttons = [MDFlatButton(text="Cancel", text_color=self.theme_cls.primary_color, on_release = self.close_dialog),
MDRectangleFlatButton(text="Ok", text_color=self.theme_cls.primary_color, on_release = self.ok_dialog),],
)
self.dialog.open()
def close_dialog(self, obj):
# Close box
self.dialog.dismiss()
def ok_dialog(self, obj):
self.dialog.dismiss()
self.root.ids.my_label.text = "ok 2toy"
MainApp().run()
|
cs |
abc.kv
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
MDBoxLayout:
orientation: 'vertical'
MDScreen:
MDRectangleFlatButton:
text: "alert popup"
pos_hint: {'center_x': .5, 'center_y': .5}
on_release: app.show_alert_dialog()
MDLabel:
id: my_label
text: "Some Stuff"
pos_hint: {'center_x': .95, 'center_y': .4}
|
cs |
main.py
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
|
from kivy.lang import Builder
from kivymd.app import MDApp
from kivymd.uix.datatables import MDDataTable
from kivymd.uix.screen import Screen
from kivy.metrics import dp
class MainApp(MDApp):
def build(self):
#define screen
screen = Screen()
#define Table
table = MDDataTable(pos_hint={'center_x': 0.5,'center_y': 0.5}, size_hint = (0.9, 0.6), check=True,
column_data=[("first name", dp(30)),("last name", dp(30)),("email", dp(30)),("hp", dp(30))],
row_data=[("2","toy","2toy@2toy.net",'010-1111-1234'),("lee","jae","lee@2toy.net",'010-3333-1321')])
#Bind the table
table.bind(on_check_press=self.checked)
table.bind(on_row_press=self.row_checked)
# self.theme_cls.theme_style = "Dark"
self.theme_cls.theme_style = "Light"
self.theme_cls.primary_palette = "BlueGray"
# return Builder.load_file('abc.kv')
screen.add_widget(table)
#add table widget
return screen
#Functionfor check presses
def checked(self, instance_table, current_row):
print(instance_table, current_row)
#Function for
def row_checked(self, instance_table, instance_row):
print(instance_table, instance_row)
MainApp().run()
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
|
from kivy.lang import Builder
from kivymd.app import MDApp
from kivymd.uix.datatables import MDDataTable
from kivymd.uix.screen import Screen
from kivy.metrics import dp
class MainApp(MDApp):
def build(self):
#define screen
screen = Screen()
#define Table
table = MDDataTable(pos_hint={'center_x': 0.5,'center_y': 0.5},
size_hint=(0.9, 0.6),
check=True,
use_pagination=True,
rows_num=3,
pagination_menu_height='240dp',
pagination_menu_pos = 'auto',
background_color=[1,0,0,.5],
column_data=[("first name", dp(30)),("last name", dp(30)),("email", dp(30)),("hp", dp(30))],
row_data=[
("2","toy","2toy@2toy.net",'010-1111-1234'),
("lee","jae","lee@2toy.net",'010-3333-1321'),
("lee","jae","lee@2toy.net",'010-3333-1321'),
("lee","jae","lee@2toy.net",'010-3333-1321'),
("lee","jae","lee@2toy.net",'010-3333-1321'),
("lee","jae","lee@2toy.net",'010-3333-1321'),
("lee","jae","lee@2toy.net",'010-3333-1321'),
("lee","jae","lee@2toy.net",'010-3333-1321'),
("lee","jae","lee@2toy.net",'010-3333-1321'),
("lee","jae","lee@2toy.net",'010-3333-1321'),
("lee","jae","lee@2toy.net",'010-3333-1321'),
("lee","jae","lee@2toy.net",'010-3333-1321'),
],)
#Bind the table
table.bind(on_check_press=self.checked)
table.bind(on_row_press=self.row_checked)
# self.theme_cls.theme_style = "Dark"
self.theme_cls.theme_style = "Light"
self.theme_cls.primary_palette = "BlueGray"
# return Builder.load_file('abc.kv')
screen.add_widget(table)
#add table widget
return screen
#Functionfor check presses
def checked(self, instance_table, current_row):
print(instance_table, current_row)
#Function for
def row_checked(self, instance_table, instance_row):
print(instance_table, instance_row)
MainApp().run()
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
|
from kivy.lang import Builder
from kivymd.app import MDApp
import sqlite3
class MainApp(MDApp):
def build(self):
self.theme_cls.theme_style = "Dark"
# self.theme_cls.theme_style = "Light"
self.theme_cls.primary_palette = "BlueGray"
#create database or connect to one
conn = sqlite3.connect('first_db.db')
#create a cursor
c = conn.cursor()
#create a table
c.execute("create table if not exists customers(name text)")
conn.commit()
conn.close()
return Builder.load_file('abc.kv')
def submit(self):
# create database or connect to one
conn = sqlite3.connect('first_db.db')
# create a cursor
c = conn.cursor()
# create a table
c.execute("insert into customers values (:first)",{
'first': self.root.ids.word_input.text,
})
self.root.ids.word_label.text = f'{self.root.ids.word_input.text} Added'
self.root.ids.word_input.text = ''
conn.commit()
conn.close()
def show_records(self):
# create database or connect to one
conn = sqlite3.connect('first_db.db')
# create a cursor
c = conn.cursor()
# create a table
c.execute("select * from customers")
records = c.fetchall()
word = ''
# loop thru records
for record in records:
word = f'{word}\n{record[0]}'
self.root.ids.word_label.text = f'{word}'
conn.commit()
conn.close()
MainApp().run()
|
cs |
abc.kv
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
MDFloatLayout:
BoxLayout:
orientation: "vertical"
size: root.width, root.height
Label:
id: word_label
text_size: self.size
halign: "center"
valign: "middle"
text: "enter a name"
font_size: 32
TextInput:
id: word_input
multiline: False
size_hint: (1, .5)
Button:
size_hint: (1, .5)
font_size: 32
text: "Submit Name"
on_press: app.submit()
Button:
size_hint: (1, .5)
font_size: 32
text: "Submit Records"
on_press: app.show_records()
|
cs |
mysql 연동
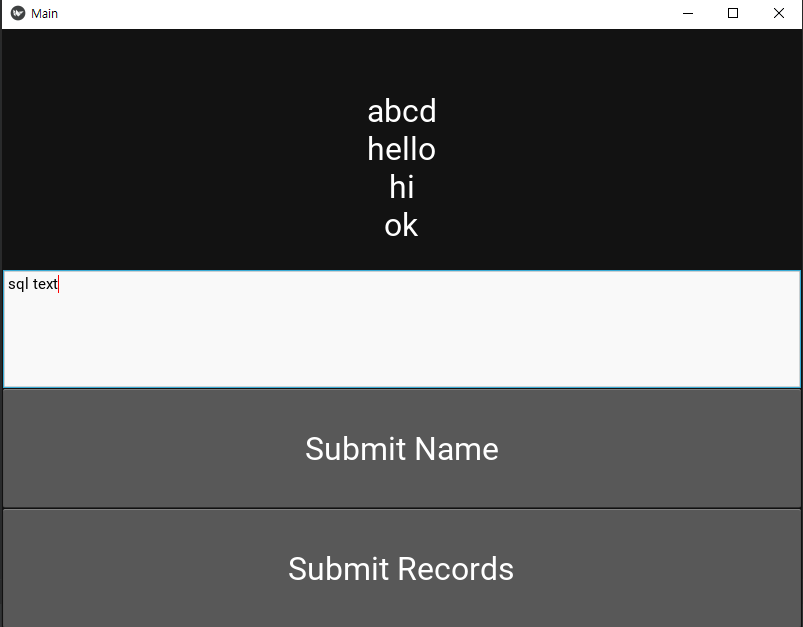
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
|
import pymysql
from kivy.lang import Builder
from kivymd.app import MDApp
class MainApp(MDApp):
def con(self):
host = "abc.net" # mysql주소
user = "abc" # mysql id
pw = "abcedfg" # mysql password
db = "sample" # mysql database
self.conn = pymysql.connect(host=host, user=user, password=pw, db=db, charset='utf8')
########################### 테이블이 없는경우 새로 생성 ###########################
try:
with self.conn.cursor() as curs:
curs.execute("""
CREATE TABLE sampleTable (or_no varchar(255) NOT NULL PRIMARY KEY
) DEFAULT CHARSET=utf8
""")
self.conn.commit() # sql문 실행
print('db 테이블생성완료')
except:
pass
###############################################################################
return self.conn
def build(self):
self.theme_cls.theme_style = "Dark"
# self.theme_cls.theme_style = "Light"
self.theme_cls.primary_palette = "BlueGray"
self.con() # sql 정보 불러오기
return Builder.load_file('abc.kv')
def submit(self):
self.conn = self.con()
try:
with self.conn.cursor() as curs:
values = (self.root.ids.word_input.text,)
sql = 'insert into sampletable(or_no) value(%s)'
curs.execute(sql, values)
self.conn.commit() # sql문 실행
print('db 내용적용 완료')
except:
pass
self.root.ids.word_label.text = f'{self.root.ids.word_input.text}'
self.root.ids.word_input.text=''
def show_records(self):
self.conn = self.con()
try:
with self.conn.cursor() as curs:
sql = 'select * from sampletable'
curs.execute(sql)
rs = curs.fetchall()
word = ''
for record in rs:
word = f'{word}\n{record[0]}'
self.root.ids.word_label.text = f'{word}'
except:
pass
MainApp().run()
|
cs |
abc.kv
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
MDFloatLayout:
BoxLayout:
orientation: "vertical"
size: root.width, root.height
Label:
id: word_label
text_size: self.size
halign: "center"
valign: "middle"
text: "enter a name"
font_size: 32
TextInput:
id: word_input
multiline: False
size_hint: (1, .5)
Button:
size_hint: (1, .5)
font_size: 32
text: "Submit Name"
on_press: app.submit()
Button:
size_hint: (1, .5)
font_size: 32
text: "Submit Records"
on_press: app.show_records()
|
cs |
'파이썬 (pythoon)' 카테고리의 다른 글
[파이썬] python 간단 계산기 코딩 (0) | 2023.02.09 |
---|---|
[파이썬] pyqt5 ui 기본소스 (0) | 2022.01.03 |
[파이썬] 가상환경 venv 설정 (터미널) (1) | 2021.10.10 |
[pyqt5 designer] 파이썬 GUI 화면 기본 구성 / 큐티 디자이너 실행 방법 / python 'ui' setup (0) | 2021.10.05 |
[pyhton] tkinter / Scrollbar / Listbox / 리스트박스 / 스크롤바 / 연결하기 (0) | 2021.07.17 |